Generate a QR Code
Creates a QR code image. You must use a PUBLIC KEY for this request.
Request
<?php
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => "https://api-us1.stannp.com/v1/qrcode/create?api_key={API_KEY}",
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => "",
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 0,
CURLOPT_FOLLOWLOCATION => true,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => "POST",
CURLOPT_POSTFIELDS => array(
'size' => "300",
'data' => "hello+world"
),
));
$response = curl_exec($curl);
curl_close($curl);
print_r($response);
?>
Response
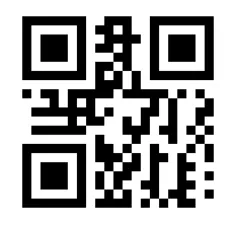
Merge PDF files
Merge multiple PDF files into a single file.
Request
<?php
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => "https://api-us1.stannp.com/v1/pdf/merge?api_key={API_KEY}",
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => "",
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 0,
CURLOPT_FOLLOWLOCATION => true,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => "POST",
CURLOPT_POSTFIELDS => array(
'files[0]' => "https://file1.pdf",
'files[1]' => "https://file2.pdf"
),
));
$response = curl_exec($curl);
curl_close($curl);
print_r($response);
?>
Response
{ "success": true, "data": "https:\/\/merged-file.pdf" }
Get templates
Get the templates created on your account.
Request
<?php
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => "https://api-us1.stannp.com/v1/templates/list?api_key={API_KEY}",
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => "",
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 0,
CURLOPT_FOLLOWLOCATION => true,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => "POST",
CURLOPT_POSTFIELDS => array(
),
));
$response = curl_exec($curl);
curl_close($curl);
print_r($response);
?>
Response
{ "success": true, "data": [ { "id": "0", "template_name": "Template name 1", "size": "US-letter", "duplex": "1" }, { "id": "1", "template_name": "Template name 2", "size": "A6", "duplex": "1" } ] }